Integration
A comprehensive guide to InviteReferrals Flutter SDK Integration helps you connect InviteReferrals with iOS and Android applications built using Flutter. Once integrated, the SDK enables you to run referral campaigns in InviteReferrals.
Read this guide to learn the basic integration of the referral campaign, how to track events, how to display a welcome message, and other important information.
Follow this guide to integrate your Flutter app with the InviteReferrals plugin.
Step 1: Add InviteReferrals Library to your Project
flutter pub add flutter_invitereferrals
Step 2: Link the library to your Project
flutter pub get
Step 3: Cocoapods (For iOS Apps)
cd ios && pod install && cd ..
Plugin Initialization
After installing the plugin, use the following platform-specific configuration.
ANDROID
-
For flutter_invitereferrals: ^1.2.0 & below use below configurations
Configure your AndroidManifest.xml
<manifest….>
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.CHANGE_NETWORK_STATE" />
<uses-permission android:name="android.permission.CHANGE_WIFI_STATE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.FOREGROUND_SERVICE" />
<application>
<meta-data
android:name="invitereferrals_bid"
android:value="10xxx" />
<meta-data
android:name="invitereferrals_bid_e"
android:value="C2C3xxxxxxxxxxxxxxxxxx" />
</application>
</manifest>
Note
In the above example, Dummy Brand ID and Encryption keys shown. Kindly login your IR_account to see your credentials.
Application Class initialization
A. If you have your own Application class, Then follow this step. Otherwise skip this step and follow Step B.
Import this statement
import com.flutter.invitereferrals.InviteReferralsPlugin;
Call this method in the onCreate() method.
InviteReferralsPlugin.register(this);
B. If you don’t have your Application class. Then add this line to your manifest.xml.
android:name="com.invitereferrals.invitereferrals.InviteReferralsApplication"
-
For flutter_invitereferrals: ^1.2.1 & later use below configurations
Step 1: Configure your app’s AndroidManifest.xml file
<manifest….>
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
………………………..
………………………..
</manifest>
Step 2: Initialize the InviteReferrals plugin
a) Create a new application class in your app if it's not created otherwise skip this step.
import android.app.Application;
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
}
}
import android.app.Application
class MyApplication : Application() {
override fun onCreate() {
super.onCreate()
}
}
Now register your application class in your app’s androidmanifest.xml file
<application
android:name=".MyApplication"
<!-- ... -->
</application>
The app directory will look like this.
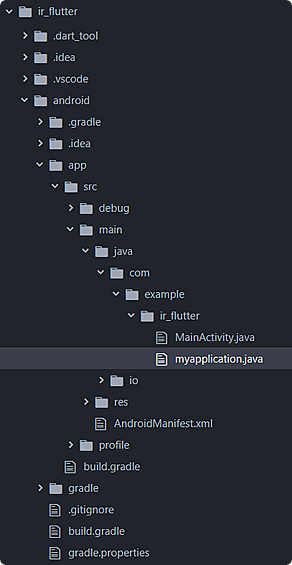
b) Import statement.
import com.flutter.invitereferrals.InviteReferralsPlugin;
import com.flutter.invitereferrals.InviteReferralsPlugin
c) Call the mentioned method in the onCreate() method.
private int INVITEREFERRALS_BRAND_ID = #####;
private String INVITEREFERRALS_BRAND_SECRET_KEY = "################################";
@Override
public void onCreate() {
super.onCreate();
InviteReferralsPlugin.register(this, INVITEREFERRALS_BRAND_ID, INVITEREFERRALS_BRAND_SECRET_KEY);
}
private val INVITEREFERRALS_BRAND_ID = #####
private val INVITEREFERRALS_BRAND_SECRET_KEY = "################################"
override fun onCreate() {
super.onCreate()
InviteReferralsPlugin.register(this, INVITEREFERRALS_BRAND_ID, INVITEREFERRALS_BRAND_SECRET_KEY)
}
iOS
Configure info.plist file
To configure your info.plist, go to iOS folder inside your Flutter Project Folder and open your iOS project into Xcode by double click on .xcworkspace file and once your flutter iOS project is open into Xcode Goto info.plist, open info.plist file as source code (right-click on info.plist and click on Open as >> Source code) and add the following code in it.
<key>CFBundleURLTypes</key>
<array>
<dict>
<key>CFBundleURLName</key>
<string>$(PRODUCT_BUNDLE_IDENTIFIER)</string>
<key>CFBundleURLSchemes</key>
<array>
<string>”yourURLscheme comes here”</string>
</array>
</dict>
</array>
<key>LSApplicationQueriesSchemes</key>
<array>
<string>whatsapp</string>
<string>fb</string>
<string>twitter</string>
</array>
OR
You can simply open the 'info.plist' file as 'Property List' and add the keys which work the same as above.
- Add a new row again and set up a URL Types item by means of adding a new item. Expand the URL Types key, then expand Item 0, and add a new item, URL Identifier . Fill in 'appScheme' for Item 0 of URL schemes and your company identifier for the URL Identifier. Once done, your file should resemble the image provided below.

- Add a new row again and set up a LSApplicationQueriesSchemes as an Array. Fill in 'whatsapp' for Item 0 , 'fb' for item 1 and 'twitter' for item 2 as illustrated in the image provided below.

Import Header File
Objective-C:
Import the InviteReferrals header file in each file in which the SDK function is to be accessed as given below.
Swift:
If your project IOS platform is in Swift language then you have to create a Bridging-Header.h file.
In this file you can import Invitereferrals SDK to use it in your project's swift files. To do so add a new header file and name it as per the following format. YOUR_FLUTTER_IOS_PROJECT_NAME-Bridging-Header.h
For example, if your project name is Runner. Then the header file name will be Runner-Bridging-Header.h. Now add the following import statement in YOUR_FLUTTER_IOS_PROJECT_NAME-Bridging-Header.h for accessing SDK Classes.
Note
Make sure that the path of bridge-header.h file is included in build settings under 'Swift compiler-code generation' as: Objective C bridging header: YOUR_FLUTTER_IOS_PROJECT_NAME/YOUR_FLUTTER_IOS_PROJECT_NAME-Bridging-Header.h
#import "InviteReferrals.h"
#import "InviteReferrals.h"
Initialize SDK into the App Delegate file
Initialize the SDK in didFinishLaunchingWithOptions function.
[[InviteReferrals sharedInstance] setupWithBrandId: BRANDID encryptedKey: @"ENCRYPTEDKEY"];
//Example
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[GeneratedPluginRegistrant registerWithRegistry:self];
// Override point for customization after application launch.
[InviteReferrals sharedInstance] setupWithBrandId: 10XXX encryptedKey: @"C2C3B406726C2F12F440XXXXXXXXXXXX"];
return [super application:application didFinishLaunchingWithOptions:launchOptions];
}
InviteReferrals.sharedInstance().setup(withBrandId: BRANDID, encryptedKey: "ENCRYPTEDKEY")
//Example
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
InviteReferrals.sharedInstance().setup(withBrandId: 10XXX, encryptedKey: "C2C3B406726C2F12F440XXXXXXXXXXXX”)
return true
}
Note
In the above example, Dummy Brand ID and Encryption keys shown. Kindly login your IR_account to see your credentials.
Add the following functions in your AppDelegate file. openURL function that will check the deep linking and open your app from the URL Scheme.
[[InviteReferrals sharedInstance] openUrlWithUrl: url];
// Example (Deep Link)
-(BOOL)application:(UIApplication *)app openURL:(NSURL *)url options:(NSDictionary<UIApplicationOpenURLOptionsKey,id> *)options {
[[InviteReferrals sharedInstance] openUrlWithUrl: url];
return nil;
}
[[InviteReferrals sharedInstance] continueUserActivityWith: userActivity];
//Example (Universal Links)
-(BOOL)application:(UIApplication *)application continueUserActivity:(NSUserActivity *)userActivity restorationHandler:(void (^)(NSArray<id<UIUserActivityRestoring>> * _Nullable))restorationHandler {
[[InviteReferrals sharedInstance] continueUserActivityWith: userActivity];
return YES;
}
InviteReferrals.sharedInstance().openUrl(with: url)
//Example (Deep Link)
func application(_ app: UIApplication, open url: URL, options: [UIApplication.OpenURLOptionsKey : Any] = [:]) -> Bool {
InviteReferrals.sharedInstance().openUrl(with: url)
return true
}
InviteReferrals.sharedInstance().continueUserActivity(with: userActivity)
//Example (Universal Links)
func application(_ application: UIApplication, continue userActivity: NSUserActivity, restorationHandler: @escaping ([UIUserActivityRestoring]?) -> Void) -> Bool {
InviteReferrals.sharedInstance().continueUserActivity(with: userActivity)
return true
}
Import inviteReferrals Package
Now in your code import the inviteReferrals package
import 'package:flutter_invitereferrals/flutter_invitereferrals.dart';
Updated 9 months ago