Campaign Screen
Referral Campaign Screen
The sharing screen appears once the user logins into the REFERRAL campaign. The screen enables him to refer the brand with his friends or acquaintances so that he could earn points and rewards for referring. This section will help you to understand how to launch the campaign screen or the login screen in your app using the InviteReferrals SDK.
Use the code mentioned below in order to activate the referral screen: -
InviteReferrals.sharedInstance().campaign(IRCampaignInfo, userInfo: IRUserInfo) { (campaignCallback:[AnyHashable : Any]?) in
}
[[InviteReferrals sharedInstance] campaign: IRCampaignInfo userInfo: IRUserInfo campaignCompletion:^(NSDictionary *campaignCallback) {
}];
Parameters
The parameters used in the above function are as follows: -
Parameter Name | Description | Value |
---|---|---|
name (string) | customer's name | abc |
email (string) | customer's email id | [email protected] |
mobileNo (string) | customer's mobile number | 9812XXXXXX |
subscriptionID (string) | customer's subscriptionID (Optional) | nil |
customValueOne (string) | Any extra value you wanted to receive (Optional) | Mumbai |
customValueTwo (string) | Any extra value you wanted to receive (Optional) | Maharashtra |
campaignID (Integer) | ID of the campaign | 10XXX or 0 (for default Campain if set in dashboard) |
templateID (Integer) | ID of the UI template | 0 (for default UI) or 1 |
When you call the above method in your app, then this function either loads the login screen or the campaign screen according to the value you have passed.
Login Screen
This screen will get loaded in case you don't pass user data in the function as shown in the example mentioned below: -
let campaignData = IRCampaignInfo()
campaignData.campaignID = 0
campaignData.templateID = 0
InviteReferrals.sharedInstance().campaign(campaignData, userInfo: nil) {
(campaignCallback:[AnyHashable : Any]?) in
print("campaign callback response = \(String(describing: campaignCallback))")
}
IRCampaignInfo *campaignData = [[IRCampaignInfo alloc] init];
campaignData.campaignID = 0;
campaignData.templateID = 0;
[[InviteReferrals sharedInstance] campaign: campaignData userInfo: nil campaignCompletion:^(NSDictionary *campaignCallback) {
NSLog(@"campaign callback response = %@", campaignCallback);
}];
You will get the following screen as an output (screenshot provided below). It's the default UI of the login screen of InviteReferrals iOS SDK.
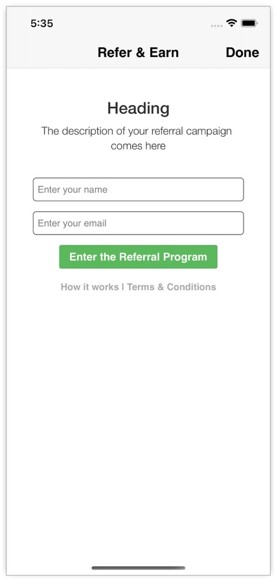
Some parts of the UI are customizable like the heading’s text, color, done button text, font etc. Here all customizations are done from IR_UserResources.plist file.
If you want to change the content of the login page, then you can change it from the IR dashboard.
Go to Campaigns → Open Referral Portal Section from Options Menu → Login Screen. From here you can change the content as per your requirements and update it. Your updated content will reflect from the next app launch onwards.
REMEMBER
On the login screen, the Name & Email Address fields are mandatory fields. You can also opt to add the Mobile Number field as per your requirement from the Login Screen section in the IR dashboard.
Campaign Screen
This screen will get loaded in case you pass user data in the function as shown in the example provided below. The sharing screen appears once the user logins into the REFERRAL campaign. The screen enables the user to refer the brand to his/her friends or acquaintances so that he/she can earn points and rewards for referring.
let campaignData = IRCampaignInfo()
campaignData.campaignID = 0
campaignData.templateID = 0
let userData = IRUserInfo()
userData.name = "abc"
userData.email = "[email protected]"
userData.mobileNo = "9818XXXXXX"
userData.subscriptionID = nil
userData.customValueOne = "custom_val_1"
userData.customValueTwo = nil
InviteReferrals.sharedInstance().campaign(campaignData, userInfo: userData) { (campaignCallback:[AnyHashable : Any]?) in
print("campaign callback response = \(String(describing: campaignCallback))")
}
IRCampaignInfo *campaignData = [[IRCampaignInfo alloc] init];
campaignData.campaignID = 0;
campaignData.templateID = 0;
IRUserInfo *userData = [[IRUserInfo alloc] init];
userData.name = @"abc";
userData.email = @"[email protected]";
userData.mobileNo = @"9818XXXXXX";
userData.customValueOne = @"custom_val_1";
userData.customValueTwo = nil;
[[InviteReferrals sharedInstance] campaign: campaignData userInfo: userData campaignCompletion:^(NSDictionary *campaignCallback) {
NSLog(@"campaign callback response = %@", campaignCallback);
}];
Different types of layouts or template variants are available for the campaign screen and each layout has its own distinct design or output.
Some parts of the UI are customizable like the size of the icons, colour of the text etc. Here all customizations are done from IR_UserResources.plist file.
If you want to change the content of the campaign page, then you can do so from the IR Dashboard.
Go to Campaigns → Open Referral Portal Section from Options Menu → Sharing Screen.
From here you can change the content as per your requirements and update it. Your updated content will reflect from the next app launch onwards.
Templates In Campaign Screen
Currently we have 2 templates for the campaign screen in our InviteReferrals SDK. For Template-1, you need to pass templateID as 0 in the function. And for Template-2, you need to pass templateID as 1 in the function. These templates for the campaign screen can be customized.
NOTE
By default Template-1 UI will load so the value of template id will be set as 0.
Template 1 [Default UI]
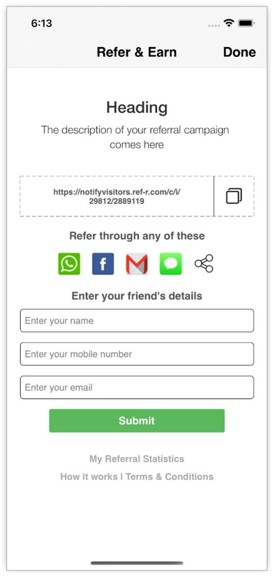
Template 2
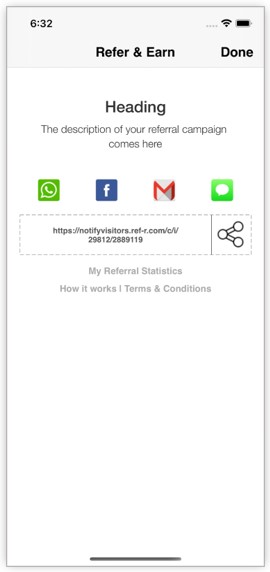
Callback / Completion Response
1. Campaign() Completion Block
As we have seen there is a compilation handler in the above described campaign() function and this will provide you a response whenever you launch the campaign screen.
Response Sample
{
"Authentication" : "success",
"message" : "Campaign UI <Login> successfully loaded.",
"type" : "1.0"
}
This completion block will give you the followings various responses as follows:
Authentication | Message | Type | Description |
---|---|---|---|
success | Campaign UI successfully loaded. Campaign UI successfully loaded. | 1.0 1.1 | When the IR campaign screen successfully launches the login UI. When the IR campaign screen successfully launches the sharing UI. |
failed | Data not found No internet connection | 2 8 | When there is no campaign data & user data available. When the internet connection not available. |
2. On Sharing Buttons Click
If you want to know on which sharing button user has clicked you get get it through a delegate method, by using InviteReferralsDelegate in your app. You need to use this delegate inside same UIViewController in which you have called campaign() function. Suppose you have called campaign() function inside ViewController, then add the delegate in your ViewController file.
class ViewController: UIViewController, InviteReferralsDelegate {
}
#import <UIKit/UIKit.h>
#import <InviteReferrals/InviteReferrals.h>
@interface ViewController : UIViewController <InviteReferralsDelegate>
@end
Now inside viewDidLoad() of your ViewController.swift/ViewController.m file, call the following method to set the delegate.
override func viewDidLoad() {
super.viewDidLoad()
InviteReferrals.sharedInstance()?.delegate = self
}
- (void)viewDidLoad {
[super viewDidLoad];
[InviteReferrals sharedInstance].delegate = self;
}
Now you can implement the following function and here you will get a callback when user clicks on any sharing options.
func inviteReferrals(onSharingIconClickCallback sharingIconClickInfo: [AnyHashable : Any]!) {
print(sharingIconClickInfo)
}
-(void)InviteReferralsOnSharingIconClickCallback:(NSDictionary *)sharingIconClickInfo {
NSLog(@"%@", sharingIconClickInfo)
}
Response Sample
{
"source":"more share option"
}
This callback will give you the following source values as follows:
Source |
---|
more share option |
SMS |
3. On Capture Lead Form Submission
If you are using a UI template in which Lead capture form is available then you can get the callback response on lead form submission by using InviteReferralsDelegate in your app. You need to use this delegate inside same UIViewController in which you have called campaign() function. Suppose you have called campaign() function inside ViewController, then add the delegate in your ViewController file.
class ViewController: UIViewController, InviteReferralsDelegate {
}
#import <UIKit/UIKit.h>
#import <InviteReferrals/InviteReferrals.h>
@interface ViewController : UIViewController <InviteReferralsDelegate>
@end
Now inside viewDidLoad() of your ViewController.swift / ViewController.m file, call the following method to set the delegate.
override func viewDidLoad() {
super.viewDidLoad()
InviteReferrals.sharedInstance()?.delegate = self
}
- (void)viewDidLoad {
[super viewDidLoad];
[InviteReferrals sharedInstance].delegate = self;
}
Now you can implement the following function and here you will get a callback when user clicks on any sharing options.
func inviteReferralsLeadSentResponseCallback(_ sentLeadResponse: [AnyHashable : Any]!) {
print(sentLeadResponse)
}
-(void)InviteReferralsLeadSentResponseCallback:(NSDictionary *)sentLeadResponse {
NSLog(@"%@", sentLeadResponse)
}
Response Sample
{
"Status":"success",
"message":"Lead capturing is successful"
}
This callback will give you the following source values as follows:
Status | Message | Description |
---|---|---|
success | Lead capturing is successful | When data is sent to the server successfully. |
failed | Incorrect name, emailID or mobile number Lead capturing is failed Something went wrong | When the user passes the wrong format of email id or mobile number. If the response from api fails. If no response is received from api. |
4. Done/Close Button Listener For Referral Screen
This delegate will be triggered whenever the referral screen of the SDK is closed using the Done / Close button in the navigation bar of the referral screen. You can achieve this by using InviteReferralsDelegate in your app You need to use this delegate inside same UIViewController in which you have called campaign() function. Suppose you have called campaign() function inside ViewController, then add the delegate in your ViewController file.
class ViewController: UIViewController, InviteReferralsDelegate {
}
#import <UIKit/UIKit.h>
#import <InviteReferrals/InviteReferrals.h>
@interface ViewController : UIViewController <InviteReferralsDelegate>
@end
Now inside viewDidLoad() of your ViewController.swift/ViewController.m file, call the following method to set the delegate.
override func viewDidLoad() {
super.viewDidLoad()
InviteReferrals.sharedInstance()?.delegate = self
}
- (void)viewDidLoad {
[super viewDidLoad];
[InviteReferrals sharedInstance].delegate = self;
}
Now you can implement the following function and here you will get a callback when user clicks on any sharing options.
func inviteReferralsOnDoneButtonAction(userInfo: [AnyHashable : Any]! = [:]) {
// Do Your stuff here …………..
}
-(void)InviteReferralsOnDoneButtonActionWithUserInfo:(NSDictionary *)userInfo {
// Do Your stuff here …………..
}
Inactive Campaign Screen
This screen is activated only when the following conditions are satisfied:-
a) Campaign’s status must be set to ‘Inactive’ in the IR dashboard.
b) Inactive Campaign Content must be entered for the campaign within its corresponding editor section (screenshot attached below).
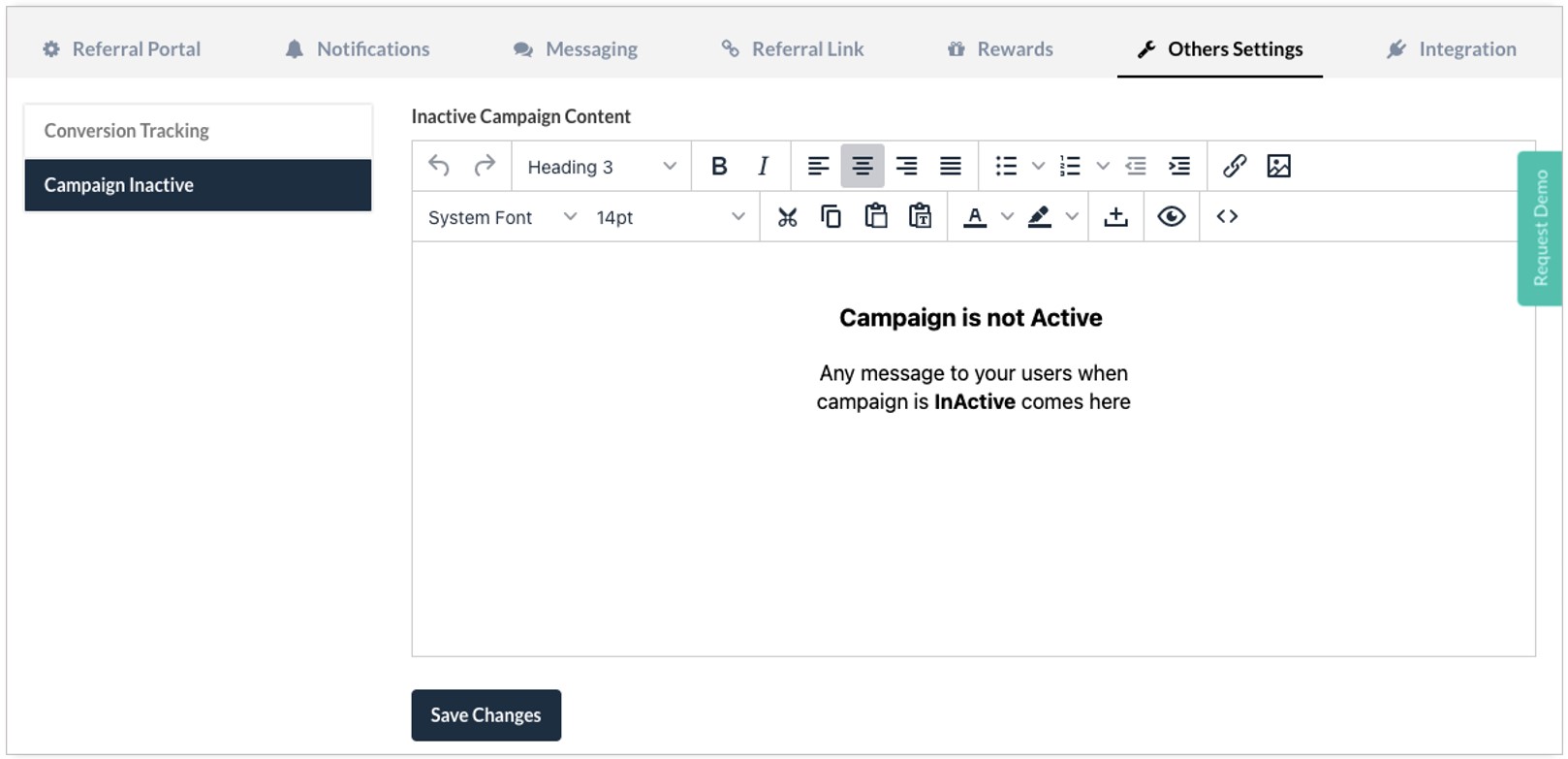
In case your campaign is set to 'Inactive' in the IR dashboard, yet you try to launch the campaign screen: -
With content in the dashboard→ then → the inactive campaign screen will be launched (sample screenshot attached below for reference).
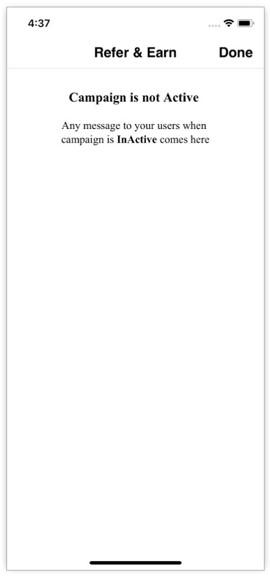
Without content in the dashboard → then → this will show you a toast with the following message “Something Went Wrong”.
Referral Statistics
This section is used to show the referral stats of the current user. In the campaign sharing screen, you will notice that there is a button titled “My Referral Statistics”. If you click on this button, a dialog box mentioning the user’s stats would appear on-screen (screenshot attached).
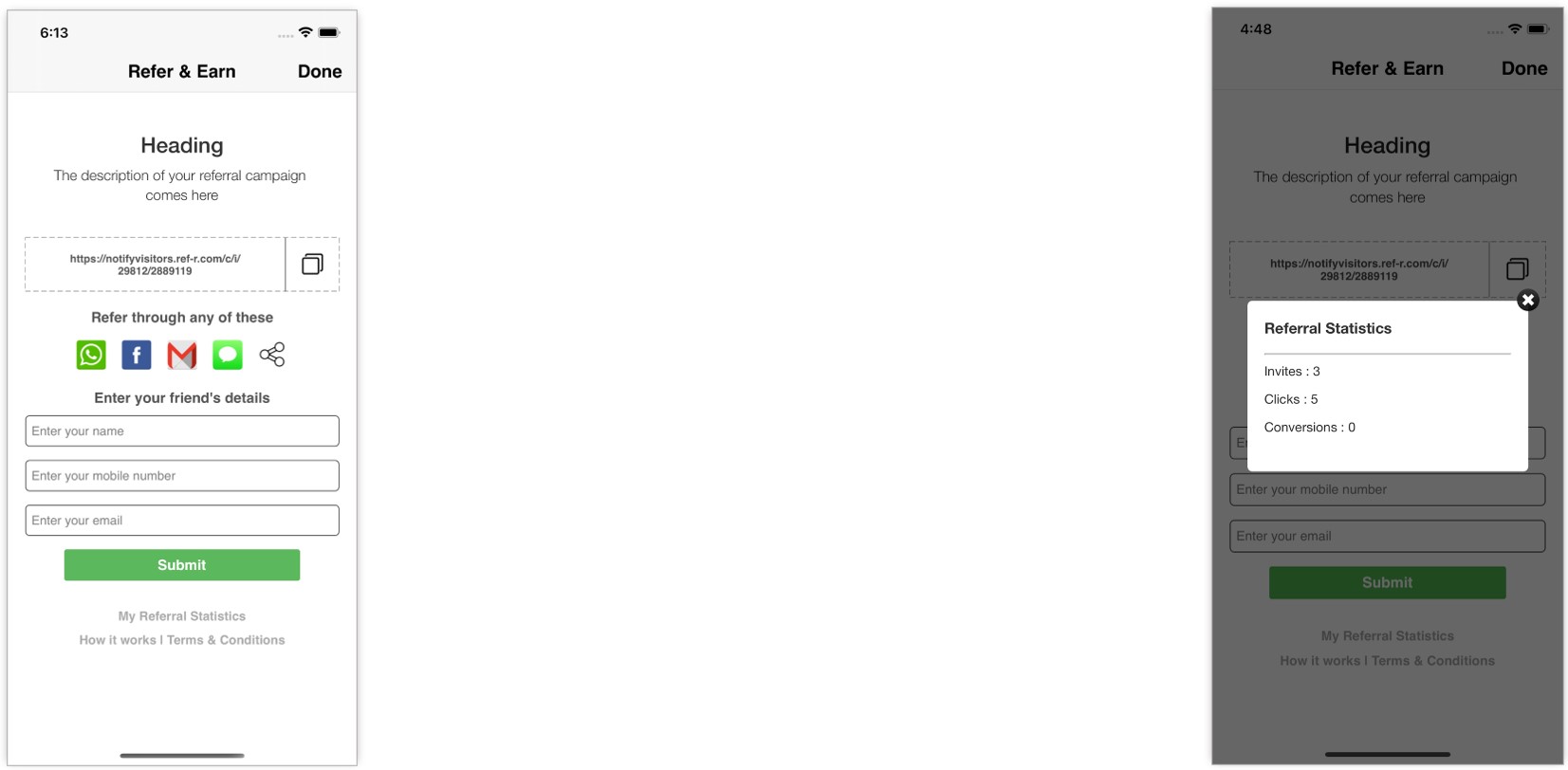
The availability of the ‘My Referral Statistics’ button on the sharing screen can be switched ON/OFF from within the IR dashboard. If you don't want to show this button on the sharing screen, then you can untick the checkbox titled ‘Show Referral Statistics’ in the IR dashboard. Find this option at Your campaign → Referral Portal → Settings.
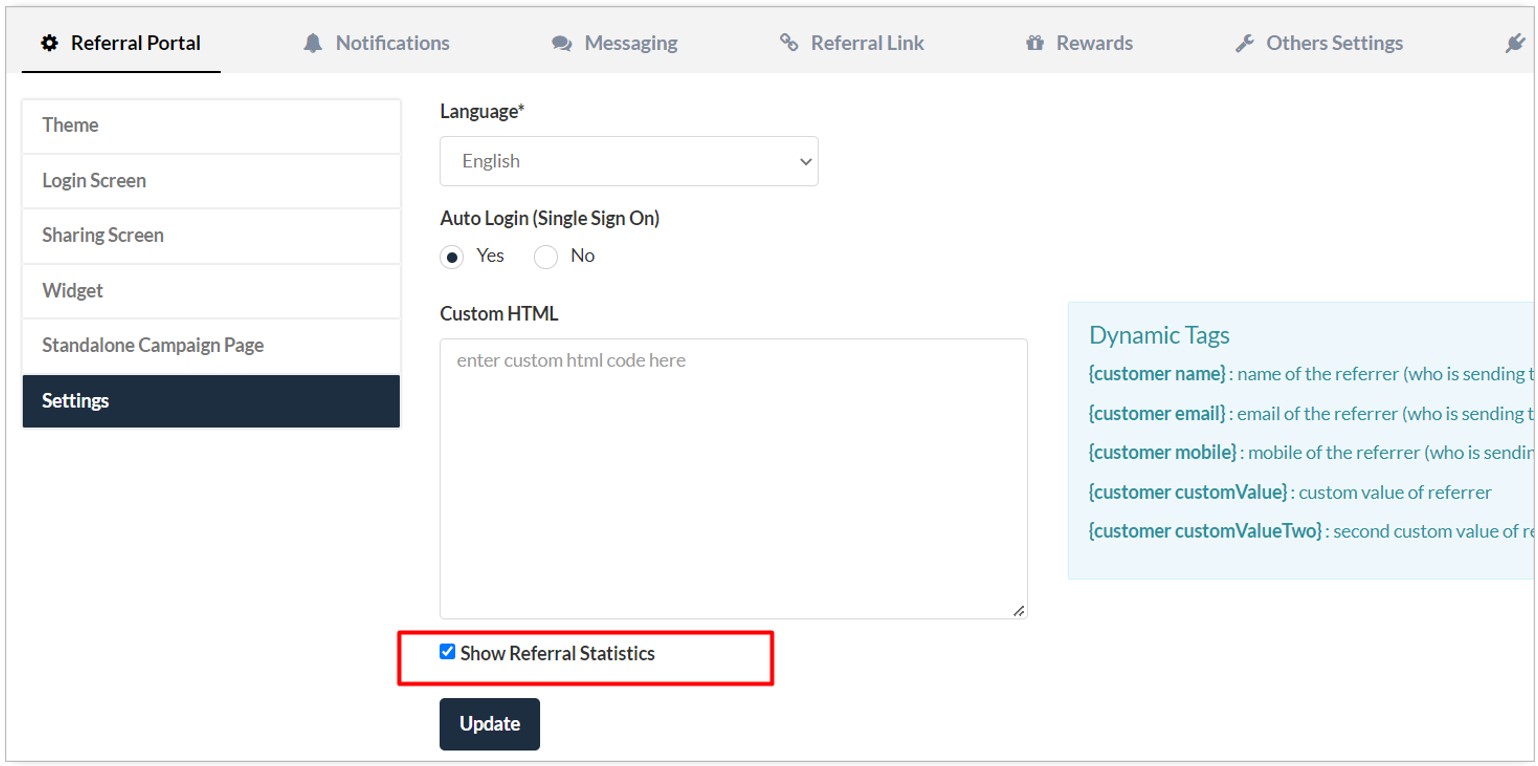
Terms & Conditions
This section is used to show the terms & conditions regarding the referral of your app to the current user. In the campaign sharing / login screen, you would notice that there exists a button titled ‘Terms & Conditions’. If you click on this button, a dialog box would appear on-screen displaying your ‘terms & conditions’ content (screenshot attached).
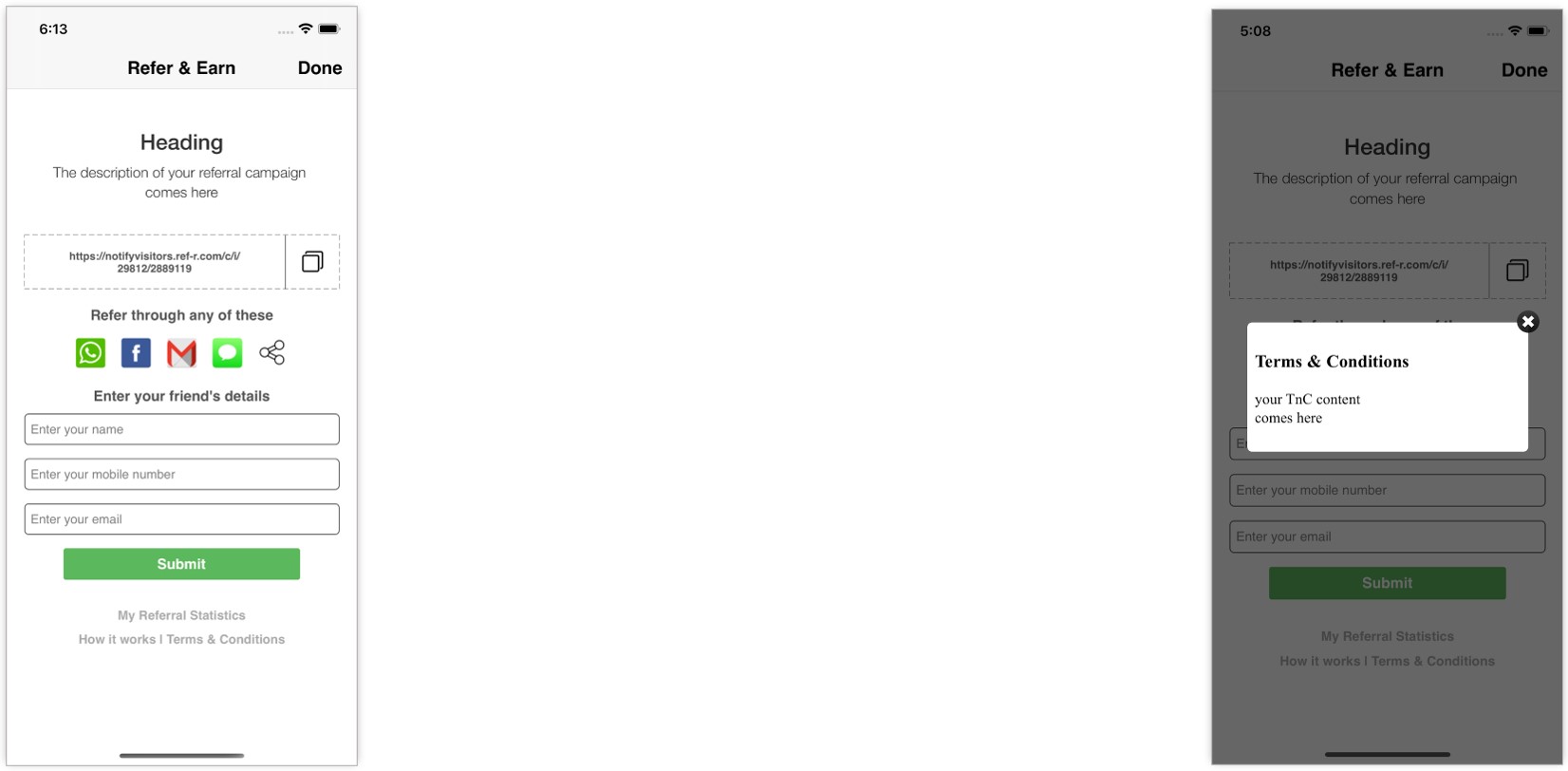
In the panel, you can enter the content at Your Campaign → Referral Portal → Settings → Bottom of the Page. If no content is available in the editor then by default Terms & Conditions button will not be displayed on the campaign screen in the app.
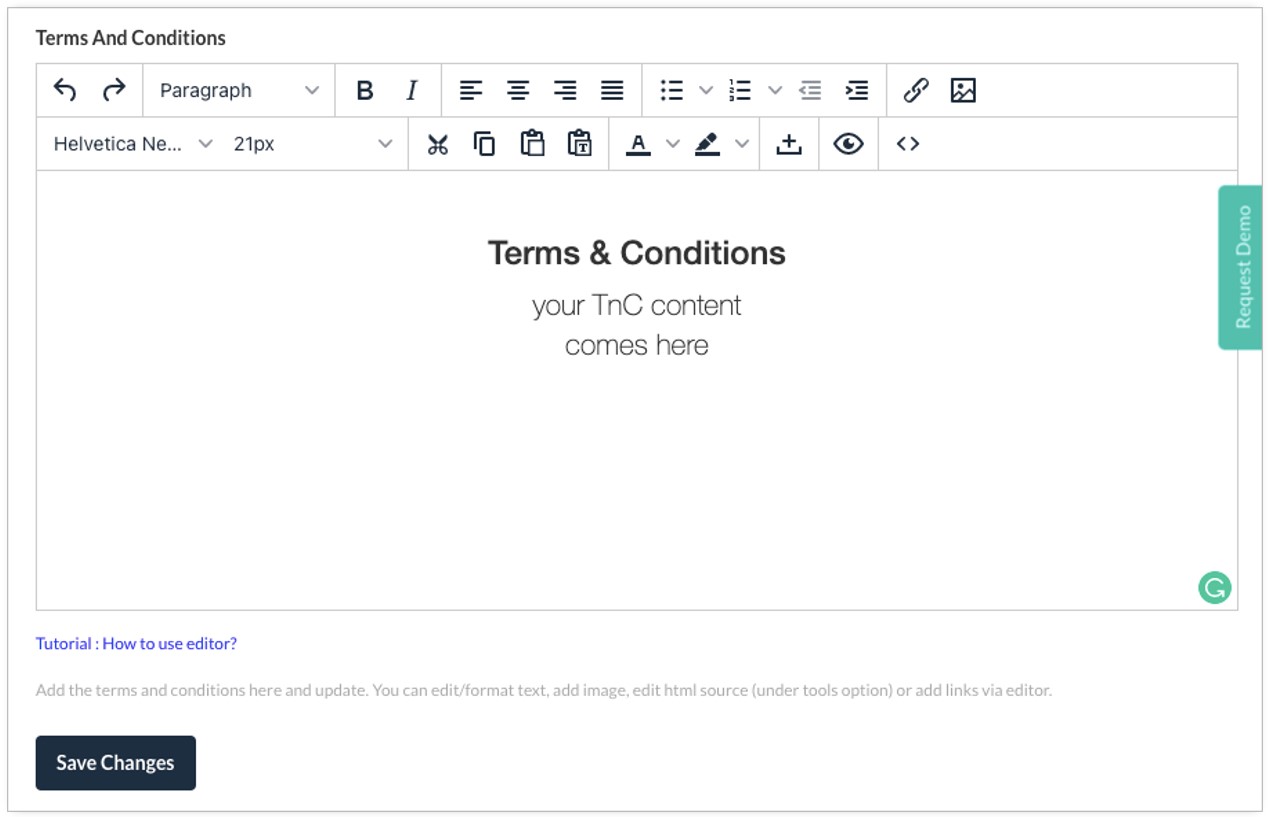
How It Works
This section outlines the functioning of the ‘How It Works’ section that is available on the screen titled ‘Refer and Earn’ which in turn gets displayed when you refer your app to any prospective user. In the campaign sharing / login screen, you would notice that there exists a button titled ‘How It Works’. If you click on this button, a dialog box would appear on-screen displaying your ‘How It Works’ content (screenshot attached below).
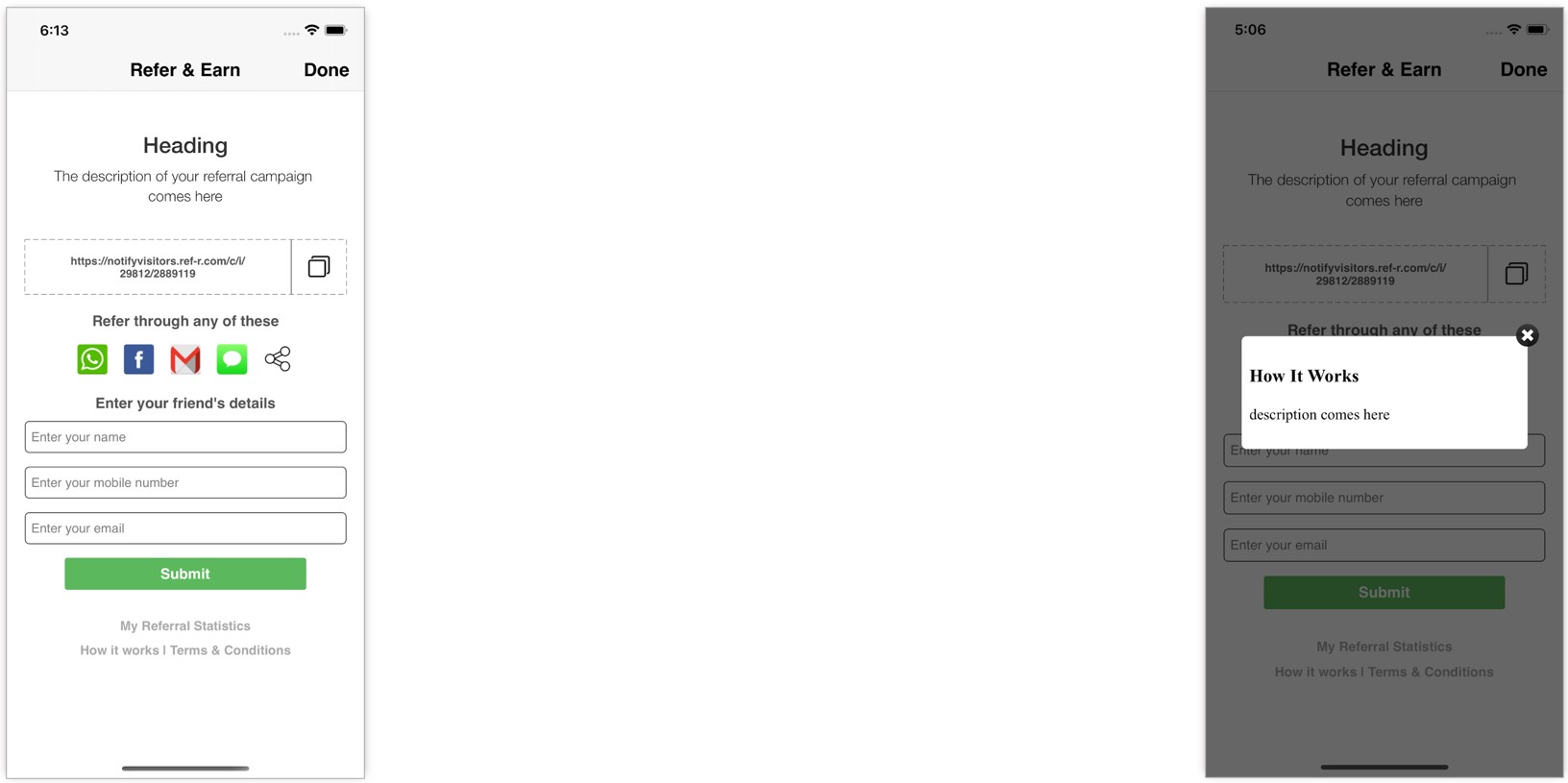
In the panel, you can enter the content at Your Campaign → Referral Portal → Settings → Middle of the Page. If no content is available in the editor, then the ‘How It Works’ button will not be displayed by default on the campaign screen within the app.
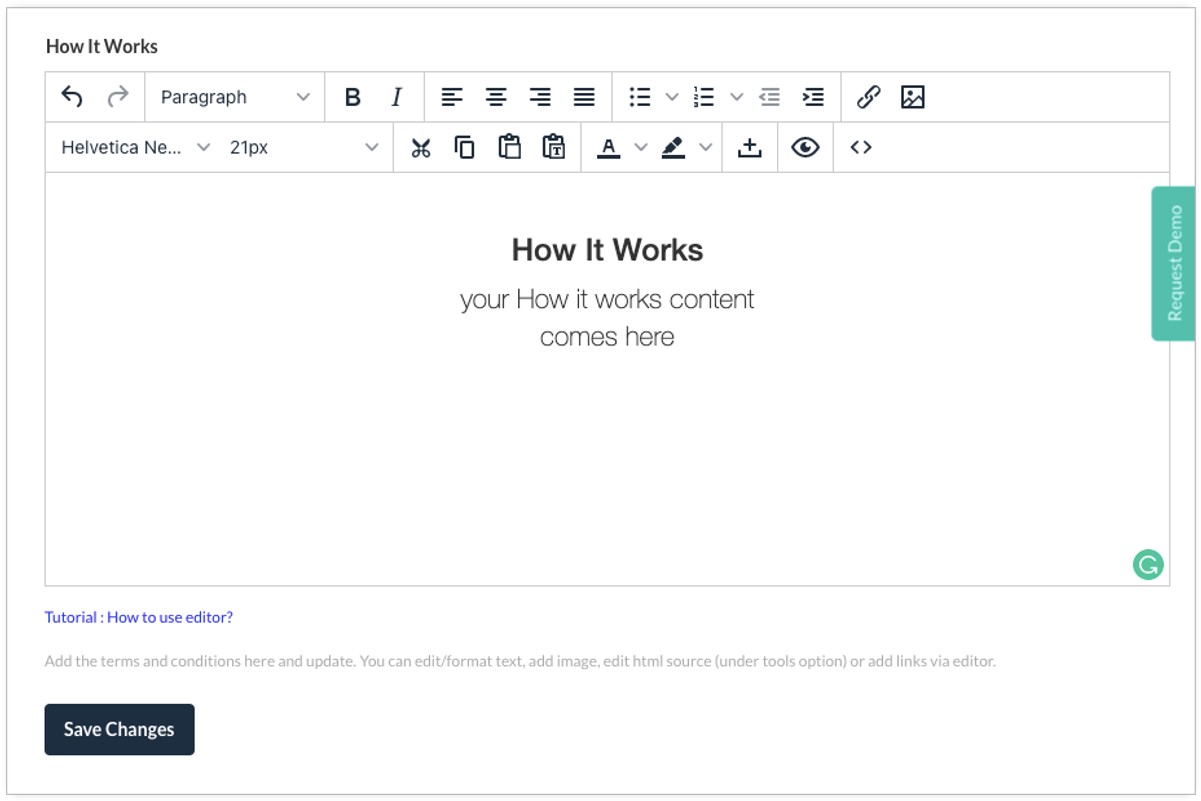
Show Campaign Invite Popup
Simply, add the following line of code in the ViewController when you want to show the referral Campaign Invite Popup.
InviteReferrals.sharedInstance().campaignPopup(forRuleName: "ruleName", campaignInfo: IRCampaignInfo, userInfo: IRUserInfo)
[[InviteReferrals sharedInstance] campaignPopupForRuleName: @"ruleName" campaignInfo: IRCampaignInfo userInfo: IRUserInfo];
Parameters
The parameters used in the above function are as follows: -
Parameter Name | Description | Value |
---|---|---|
ruleName (string) | Name of the rule to activate popup | Home referralInvite |
name (string) | customer's name | abc |
email (string) | customer's email id | [email protected] |
mobileNo (string) | customer's mobile number | 9812XXXXXX |
subscriptionID (string) | customer's subscriptionID (Optional) | nil |
customValueOne (string) | Any extra value you wanted to receive (Optional) | Mumbai |
customValueTwo (string) | Any extra value you wanted to receive (Optional) | Maharashtra |
campaignID (Integer) | ID of the campaign | 10XXX or 0 (for default Campain if set in dashboard) |
templateID (Integer) | ID of the UI template | 0 (for default UI) or 1 |
To activate the referral Campaign Invite Popup, you need to call the function mentioned above as depicted in the following example: -
let campaignData = IRCampaignInfo()
campaignData.campaignID = 0
campaignData.templateID = 0
let userData = IRUserInfo()
userData.name = "abc"
userData.email = "[email protected]"
userData.mobileNo = "9818XXXXXX"
userData.subscriptionID = nil
userData.customValueOne = "custom_val_1"
userData.customValueTwo = nil
InviteReferrals.sharedInstance().campaignPopup(forRuleName: "referralInvite", campaignInfo: campaignData, userInfo: userData)
IRCampaignInfo *campaignData = [[IRCampaignInfo alloc] init];
campaignData.campaignID = 0;
campaignData.templateID = 0;
IRUserInfo *userData = [[IRUserInfo alloc] init];
userData.name = @"abc";
userData.email = @"[email protected]";
userData.mobileNo = @"9818XXXXXX";
userData.customValueOne = @"custom_val_1";
userData.customValueTwo = nil;
[[InviteReferrals sharedInstance] campaignPopupForRuleName: @"referralInvite" campaignInfo: campaignData userInfo: userData];
Note
RuleName will be checked against campaignID.
• If user data is not passed in the function then the widget will redirect to the IR login screen.
• If campaign data is not passed in the function then the widget will not work & the following log will be printed “No Campaign Data passed from the function.”.
• Rule Name & corresponding campaignID is required.
The Campaign Invite Popup will display the content you have passed in the Login Screen Editor section in the IR dashboard. (screenshot attached)
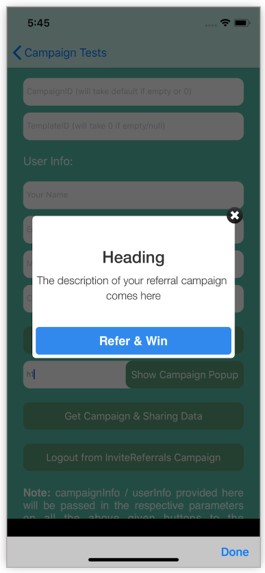
In the IR dashboard you can configure rules at Your Campaign → Integration → Mobile App.
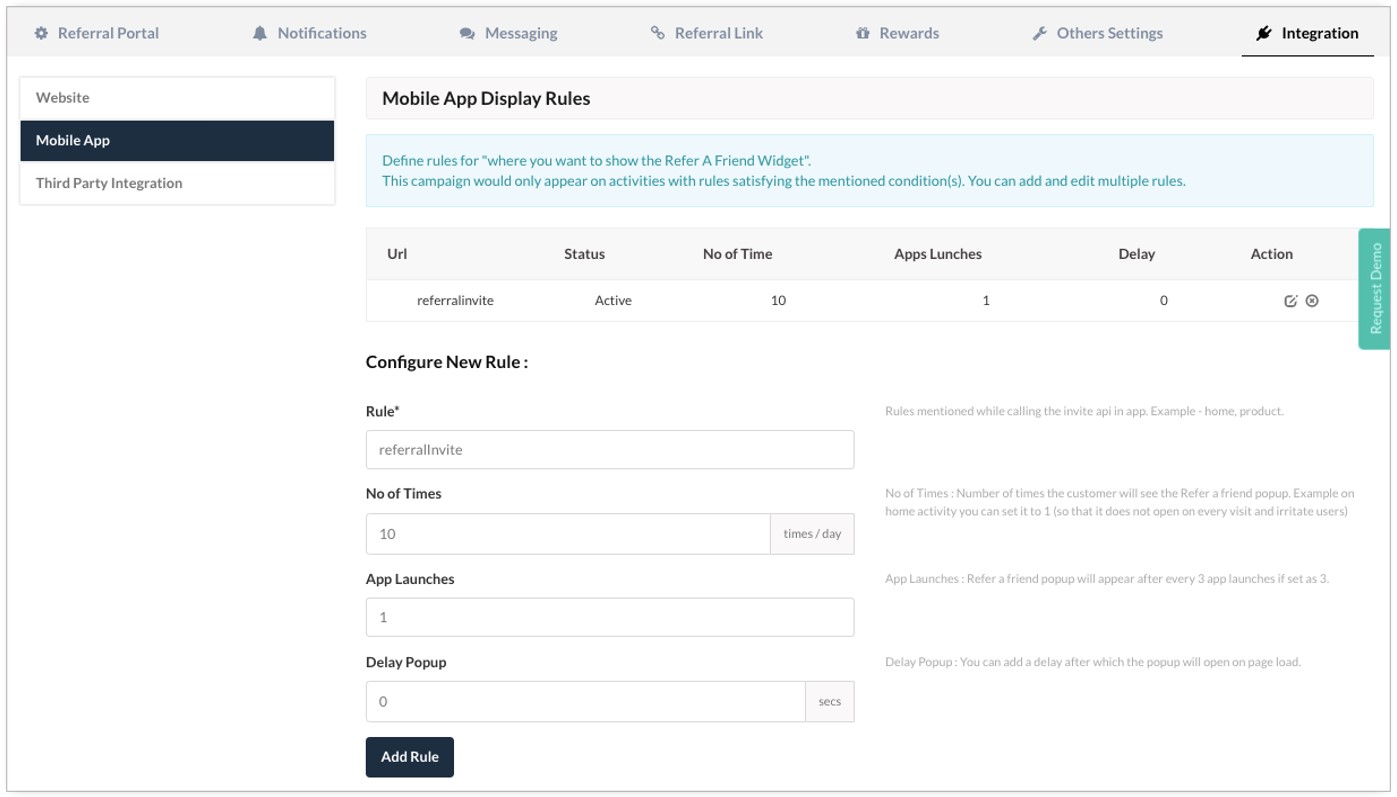
Logout (From InviteReferral Campaign)
If you want to logout user from the campaign in the same session to meet your requirement or referral flow then you can logout user from the logged in campaign by using the following method.
InviteReferrals.sharedInstance().logout()
[[InviteReferrals sharedInstance] logout];
By default, SDK automatically clears all user data on every app launch.
Create Your Own Campaign Screen
This function can be used, in case you want to design your own custom campaign screen, using our campaign data.
let campaignData = IRCampaignInfo()
campaignData.campaignID = 0
campaignData.templateID = 0
let userData = IRUserInfo()
userData.name = "abc"
userData.email = "[email protected]"
userData.mobileNo = "9818XXXXXX"
userData.subscriptionID = nil
userData.customValueOne = "custom_val_1"
userData.customValueTwo = nil
InviteReferrals.sharedInstance().getSharingDetails(with: campaignData, userInfo: userData) { (irSharingContent: [AnyHashable : Any]?) in
print("SharingContent = \(irSharingContent!)")
}
IRCampaignInfo *campaignData = [[IRCampaignInfo alloc] init];
campaignData.campaignID = 0;
campaignData.templateID = 0;
IRUserInfo *userData = [[IRUserInfo alloc] init];
userData.name = @"abc";
userData.email = @"[email protected]";
userData.mobileNo = @"9818XXXXXX";
userData.customValueOne = @"custom_val_1";
userData.customValueTwo = nil;
[[InviteReferrals sharedInstance] getSharingDetailsWithCampaignInfo:campaignData userInfo: userData sharingData:^(NSDictionary *irSharingContent) {
NSLog(@"SharingContent = %@", irSharingContent);
}];
NOTE
If you don't pass user data then this callback will provide you with only the campaign-related data. However, if you pass user data then it will provide all data i.e. campaign data along with the user data.
Response Sample With User Data
{
"sms_shareText": "Refer and win #Referral #Contests #inviteReferrals",
"twitter_shareText": "Refer and win #Referral #Contests #inviteReferrals",
"email_shareText": "<table>\r\n <tr>\r\n <td>Hi,</td>\r\n </tr>\r\n <tr>\r\n <td></td>\r\n </tr>\r\n <tr>\r\n <td>Your friend Tom, has invited you to try InviteReferrals through his referral link</td>\r\n </tr>\r\n <tr>\r\n <td></td>\r\n </tr>\r\n <tr>\r\n <td>Accept the invitation by clicking the link mentioned below.</td>\r\n </tr>\r\n <tr>\r\n <td>https://notifyvisitors.ref-r.com/c/i/29812/61236843</td>\r\n </tr>\r\n</table>",
"campaignID": "29812",
"pinterest_shareText": "Refer and win #Referral #Contests #inviteReferrals",
"shareSubject": "Explore InviteReferrals Referral Contest",
"loginScreenData": "<link rel=\"stylesheet\" href=\"https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css\"><style>body{background: transparent;}</style><div class=\"text-center\">\n <h3><br />Heading</h3>\n <p class=\"lead\">\n The description of your referral campaign \n <br>\n comes here\n </p>\n</div>",
"popup_shareText": "Refer and win #Referral #Contests #inviteReferrals",
"Authentication": "success",
"tracking_period": 30,
"Email": "[email protected]",
"linkedin__shareText": "Refer and win #Referral #Contests #inviteReferrals",
"CustomerName": "Tom",
"referral_link": "https://notifyvisitors.ref-r.com/c/i/29812/61236843",
"shareText": "Refer and win #Referral #Contests #inviteReferrals",
"referral_code": "NEERYSC3",
"shareScreenData": "<link rel=\"stylesheet\" href=\"https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css\"><style>body{background: transparent;}</style><div class=\"text-center\">\n <h3><br />Heading</h3>\n <p class=\"lead\">\n The description of your referral campaign\n <br>\n comes here\n </p>\n</div>",
"fb_shareText": {
"fbImage": "https://s3.ap-south-1.amazonaws.com/tagnpin-mumbai/images/fb_10209_29812.jpg",
"fb_shareText": "Refer and win #Referral #Contests #inviteReferrals",
"fbReferral_link": "https://notifyvisitors.ref-r.com/c/i/29812/61236843?r=fb"
},
"whatsapp_shareText": "Refer and win #Referral #Contests #inviteReferrals",
"unique_code": null
}
Response Sample Without User Data
{
"Authentication": "success",
"campaignID": 200,
"popup_shareText": "{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"shareSubject": "Explore Tagnpin Referral Contest",
"inviteMail": "Hi, Your friend {customer name}, has invited you to try Tagnpin through his referral link. Accept the invitation by clicking the link mentioned below. {referral link}",
"twitter_share_text": "{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"email_shareText": "Hi, Your friend {customer name}, has invited you to try Tagnpin through his referral link. Accept the invitation by clicking the link mentioned below. {referral link}",
"whatsapp_shareText": "Refer and win #Referral #Contests #inviteReferrals ",
"sms_shareText": "{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"twitter_shareText": "{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"linkedin_shareText": "{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"pinterest_shareText": "{referral link} Refer and win #Referral #Contests #inviteReferrals ".
"fb_shareText": {
"fbLink": "{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"fbImage": "https://s3.ap-south-1.amazonaws.com/stage-tagnpin/images/fb_49_200.jpg"
},
"loginScreenData": "<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css"><style>body{background: transparent;}</style><div class="text-center"> <h3><br />Heading</h3> <p class="lead"> The description of your referral campaign <br> comes here </p> </div>",
"shareScreenData": "<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css"><style>body{background: transparent;}</style><div class="text-center"> <h3><br />Heading</h3> <p class="lead">The description of your referral campaign <br />comes here<br /><span style="color: #ba372a;"><strong>I am from Campaign : 200</strong></span></p> </div>",
"howItWorks": "<div><img src="https://as2.ftcdn.net/v2/jpg/01/87/31/25/1000_F_187312561_kMY7AYVLSwCz6Jua7KusMMPQtb4TayFA.jpg" width="500" height="500" alt="" style="display: block; margin-left: auto; margin-right: auto;" /></div>",
"tnc": "This is Terms and Conditions section."
}
Updated over 1 year ago