IR Campaign Screen Launch
This section will help you to understand how to launch the campaign screen or the login screen in your app using the InviteReferrals SDK.
Use the code mentioned below in order to activate the referral screen: -
InviteReferralsApi.getInstance(activityContext).campaign(IRUserData.Builder userInfo, IRCampaignData.Builder campaignInfo, OnUIBuild onUIBuild)
InviteReferralsApi.getInstance(activityContext).campaign(IRUserData.Builder userInfo, IRCampaignData.Builder campaignInfo, OnUIBuild onUIBuild)
Parameters
The parameters used in the above function are as follows: -
Parameter | Description | Value |
---|---|---|
setUserName (string) | customer's name | abc |
setUserEmail (string) | customer's email id | [email protected] |
setUserMobile (string) | customer's mobile number | 9812XXXXXX |
setCustomValueOne (string) | Any extra value you wanted to receive | Mumbai |
setCustomValueTwo (string) | Any extra value you wanted to receive | Maharashtra |
setCampaignID (int) | ID of the campaign | 10XXX |
setTemplateID (int) | ID of the UI template | 0 (for default UI) or 1 |
When you call the above method in your app, then this function either loads the login screen or the campaign screen according to the value you have passed.
Login Screen
This screen will get loaded in case you don't pass user data in the function as shown in the example mentioned below: -
IRCampaignData.Builder campaignInfo = new IRCampaignData.Builder();
campaignInfo.setCampaignID(0);
campaignInfo.setTemplateID(1);
campaignInfo.build();
InviteReferralsApi.getInstance(activityContext).campaign(null, campaignInfo, new OnUIBuild() {
@Override
public void onCampaignScreen(JSONObject response) {
//do your task here
}
@Override
public void onShareIconClick(JSONObject data) {
//do your task here
}
@Override
public void onCloseButtonClick() {
//do your task here
}
@Override
public void onCaptureLeadFormSubmission(JSONObject response) {
//do your task here
}
});
val campData = IRCampaignData.Builder();
campData.setCampaignID(0);
campData.setTemplateID(1);
campData.build();
InviteReferralsApi.getInstance(activityContext).campaign(null, campData, object: OnUIBuild {
override fun onCampaignScreen(p0: JSONObject?) {
//do your task here
}
override fun onShareIconClick(p0: JSONObject?) {
//do your task here
}
override fun onCloseButtonClick() {
//do your task here
}
override fun onCaptureLeadFormSubmission(p0: JSONObject?) {
//do your task here
}
})
You will get the following screen as an output (screenshot provided below). It's the default UI of the login screen of InviteReferrals Android SDK.
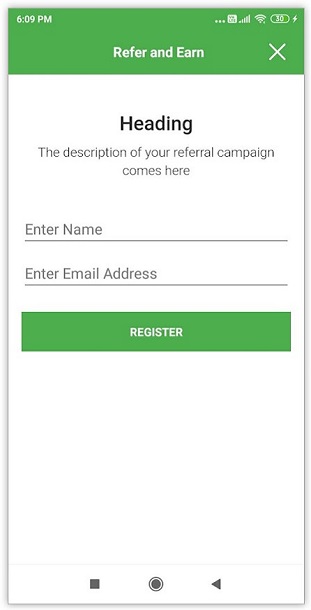
Some parts of the UI are customizable like the heading’s text, the heading’s color, the image of the cross-shaped button etc. Here all customizations are done from the various resource files like strings.xml or integers.xml, etc. For further information refer to the section titled ‘Campaign Screen UI Customization(s) & Other Configurations’.
If you want to change the content of the login page, then you can change it from the IR dashboard.
Go to Campaigns → Open Referral Portal Section from Options Menu → Login Screen. From here you can change the content as per your requirements and update it. Your updated content will reflect from the next app launch onwards.
REMEMBER
Within the login screen, the Name & Email Address fields are mandatory fields. You can also opt to add the Mobile Number field as per your requirement from the Login Screen section in the IR dashboard.
Campaign Screen
This screen will get loaded in case you pass user data in the function as shown in the example provided below. The sharing screen appears once the user logins into the REFERRAL campaign. The screen enables the user to refer the brand to his/her friends or acquaintances so that he/she can earn points and rewards for referring.
IRUserData.Builder userInfo = new IRUserData.Builder();
userInfo.setUserName(“abc”);
userInfo.setUserEmail(“[email protected]”);
userInfo.setUserMobile(“9999999999”);
userInfo.setCustomValueOne(“custom_val_1”);
userInfo.setCustomValueTwo(“custom_val_2”);
userInfo.build();
IRCampaignData.Builder campaignInfo = new IRCampaignData.Builder();
campaignInfo.setCampaignID(0);
campaignInfo.setTemplateID(0);
campaignInfo.build();
InviteReferralsApi.getInstance(activityContext).campaign(userInfo, campaignInfo, new OnUIBuild() {
@Override
public void onCampaignScreen(JSONObject response) {
//do your task here
}
@Override
public void onShareIconClick(JSONObject data) {
//do your task here
}
@Override
public void onCloseButtonClick() {
//do your task here
}
@Override
public void onCaptureLeadFormSubmission(JSONObject response) {
//do your task here
}
});
});
val userData = IRUserData.Builder();
userData.setUserName("abc");
userData.setUserEmail("[email protected]");
userData.setUserMobile("9999999999");
userData.setCustomValueOne("custom_val_1");
userData.setCustomValueTwo("custom_val_2");
userData.build();
val campData = IRCampaignData.Builder();
campData.setCampaignID(0);
campData.setTemplateID(0);
campData.build();
InviteReferralsApi.getInstance(activityContext).campaign(userData, campData, object: OnUIBuild {
override fun onCampaignScreen(p0: JSONObject?) {
//do your task here
}
override fun onShareIconClick(p0: JSONObject?) {
//do your task here
}
override fun onCloseButtonClick() {
//do your task here
}
override fun onCaptureLeadFormSubmission(p0: JSONObject?) {
//do your task here
}
})
Some parts of the UI are customizable like the size of the icons, colour of the text etc. Here all customizations are done from the various resource files such as strings.xml, integers.xml etc. For further information refer to the section titled ‘Campaign Screen UI Customization(s) & Other Configurations’.
If you want to change the content of the campaign page, then you can do so from the IR dashboard.
Go to Campaigns → Open Referral Portal Section from Options Menu → Sharing Screen. From here you can change the content as per your requirements and update it. Your updated content will reflect from the next app launch onwards.
Templates In Campaign Screen
Currently we have 2 templates for the campaign screen in IR SDK. For Template1, you need to pass templateID as 0 in the function. And for Template2, you need to pass templateID as 1 in the function. These templates for the campaign screen can be customized.
NOTE
By default, the value will be 0.
Template 1 [Default UI]
Template 2
Callback Response
The function described above will provide you with 4 different callbacks. Their responses are as follows: -
1. onCampaignScreen()
This function will provide you a response whenever the campaign screen’s loading process starts.
{
"status":"success",
"message":"Campaign UI <LOGIN> successfully loaded.",
"type":"_1_0"
}
The messages that you receive in this function are as follows: -
Status | Message | Type | Description |
---|---|---|---|
success | Campaign UI successfully loaded. Campaign UI successfully loaded. | _1_1 _1_0 | When the IR campaign screen successfully launches the login UI. When the IR campaign screen successfully launches the sharing UI. |
fail | Data not found Campaign data not found Campaign data not found Something went wrong with the following error → Something went wrong while fetching campaignID. Something went wrong while parsing with the following error → Something went wrong with error → CAMPAIGNID = ; Campaign is Inactive. Campaign id is invalid Parameter missing in response from user details. | _2_0 _2_1 _2_3 _2_2 _3_0 _3_1 _3_2 _4_0 _4_2 _4_4 _4_5 _4_6 _4_1 _4_3 _4_7 _5_0 _5_1 _6_0 _7_0 _7_1 | When there is no campaign data & user data available in the IR-SDK. When campaign data is not passed in the campaign() function. When campaign data is not available. When any exception occurred while reading campaign data from the file in the IR-SDK. Any error occurred while retrieving campaignID internally. Any error occurred while parsing campaign data internally. When any exception occurred while checking data passed in the campaign() function. When a campaign is inactive from the IR dashboard. When a campaign id passed in the campaign() function is invalid. When user stats are found missing. |
2. onShareIconClick()
This function will provide you with a response whenever any custom share icon is clicked on the sharing screen.
{
"source":"More Share Option"
}
The messages that you receive as source in this function, are as follows: -
Source |
---|
More Share Option |
Gmail |
Contact Sync |
Facebook Messenger |
SMS |
Telegram |
3. onCloseButtonClick()
This function will not provide you any response. It will only be triggered & give you the hit on the click of the close button on the campaign screen.
4. onCaptureLeadFormSubmission()
This function will provide you with a response whenever the user fills the capture lead form on the sharing screen (templateID as 0).
{
"status":"fail",
"message":"Incorrect EmailID or Mobile Number"
}
The messages you receive in this function are as follows: -
Status | Message | Description |
---|---|---|
success | Lead Capturing is Successful | When data is sent to the server successfully. |
fail | Incorrect EmailID or Mobile Number Lead Capturing is Failed Something Went Wrong | When the user passes the wrong format of email id or mobile number. If the response from API fails. If no response is received from API. If any exception occurred while sending a request to the server. |
Inactive Campaign Screen
This screen is activated only when the following conditions are satisfied: -
a. Campaign’s status must be set to ‘Inactive’ in the IR dashboard.
b. Inactive Campaign Content must be entered for the campaign within its corresponding editor section (screenshot attached below).
In case your campaign is set to 'Inactive' in the IR dashboard, yet you try to launch the campaign screen: -
- With content in the dashboard → then → the inactive campaign screen will be launched (sample screenshot attached below for reference).
- Without content in the dashboard → then → this will show you a toast with the following message “Something Went Wrong”.
Referral Statistics
This section is used to show the referral stats of the current user. In the campaign sharing screen, you will notice that there is a button titled “My Referral Statistics”. If you click on this button, a dialog box mentioning the user’s stats would appear on-screen. (screenshot attached)
The availability of the ‘My Referral Statistics’ button on the sharing screen can be switched ON/OFF from within the IR dashboard. If you don't want to show this button on the sharing screen, then you can untick the checkbox titled ‘Show Referral Statistics’ in the IR dashboard. Find this option at Your campaign → Referral Portal → Settings.
Terms & Conditions
This section is used to show the terms & conditions regarding the referral of your app to the current user. In the campaign sharing / login screen, you would notice that there exists a button titled ‘Terms & Conditions’. If you click on this button, a dialog box would appear on-screen displaying your ‘terms & conditions’ content. (screenshot attached)
In the panel, you can enter the content at Your Campaign → Referral Portal → Settings → Bottom of the Page. If no content is available in the editor then by default Terms & Conditions button will not be displayed on the campaign screen in the app.
How It Works
This section outlines the functioning of the ‘How It Works’ section that is available on the screen titled ‘Refer and Earn’ which in turn gets displayed when you refer your app to any prospective user. In the campaign sharing / login screen, you would notice that there exists a button titled ‘How It Works’. If you click on this button, a dialog box would appear on-screen displaying your ‘How It Works’ content (screenshot attached below).
In the panel, you can enter the content at Your Campaign → Referral Portal → Settings → Middle of the Page. If no content is available in the editor, then the ‘How It Works’ button will not be displayed by default on the campaign screen within the app.
Show Refer A Friend Widget
Simply, add the following line of code in the activity in which you want to show the referral program widget.
InviteReferralsApi.getInstance(activityContext).campaignPopup(String ruleName, IRUserData.Builder userInfo, IRCampaignData.Builder campInfo);
InviteReferralsApi.getInstance(activityContext).campaignPopup(String ruleName, IRUserData.Builder userInfo, IRCampaignData.Builder campInfo);
Parameters
Following are the parameters used in the function mentioned above: -
Parameter | Description | Value |
---|---|---|
ruleName (string) | Name of the rule to activate popup | home |
setUserName (string) | customer's name | abc |
setUserEmail (string) | customer's email id | [email protected] |
setUserMobile (string) | customer's mobile number | 9812XXXXXX |
setCustomValueOne (string) | Any extra value you wanted to receive | mumbai |
setCustomValueTwo (string) | Any extra value you wanted to receive | maharashtra |
setCampaignID (int) | ID of the campaign | 10XXX |
setTemplateID (int) | ID of the UI template | 0 (for default UI) or 1 |
To activate the widget, you need to call the function mentioned above as depicted in the following example: -
IRUserData.Builder userInfo = new IRUserData.Builder();
userInfo.setUserName(“abc”);
userInfo.setUserEmail(“[email protected]”);
userInfo.setUserMobile(“9090909090”);
userInfo.setCustomValueOne(null);
userInfo.setCustomValueTwo(null);
userInfo.build();
IRCampaignData.Builder campaignInfo = new IRCampaignData.Builder();
campaignInfo.setCampaignID(123);
campaignInfo.setTemplateID(1);
campaignInfo.build();
InviteReferralsApi.getInstance(activityContext).campaignPopup("home", userInfo, campaignInfo);
val campaignInfo = IRCampaignData.Builder();
campaignInfo.setCampaignID(123);
campaignInfo.setTemplateID(1);
campaignInfo.build();
val userInfo = IRUserData.Builder();
userInfo.setUserName("abc");
userInfo.setUserEmail("[email protected]");
userInfo.setUserMobile("9090909090");
userInfo.setCustomValueOne(null);
userInfo.setCustomValueTwo(null);
userInfo.build();
InviteReferralsApi.getInstance(activityContext).campaignPopup("home", userInfo, campaignInfo);
NOTE
RuleName will be checked against campaignID.
- If user data is not passed in the function then the widget will redirect to the IR login screen.
- If campaign data is not passed in the function then the widget will not work & the following log will be printed “No Campaign Data passed from the function.”;
- Rule Name & corresponding campaignID is required.
The widget will display the content you have passed in the Login Screen Editor section in the IR dashboard. (screenshot attached)
In the IR dashboard you can configure rules at Your Campaign → Integration → Mobile App.
Clear User Details
If you want to clear user data from the IR-SDK, then you can call the function mentioned below: -
InviteReferralsApi.getInstance(activityContext).clearUserDetails();
InviteReferralsApi.getInstance(activityContext).clearUserDetails()
By default, SDK automatically clears all user data on every app launch.
Create Your Own Campaign Screen
This function can be used, in case you want to design your own custom campaign screen, using our campaign data.
IRUserData.Builder userInfo = new IRUserData.Builder();
userInfo.setUserName(name);
userInfo.setUserEmail(email);
userInfo.setUserMobile(mobile);
userInfo.setCustomValueOne(null);
userInfo.setCustomValueTwo(null);
userInfo.build();
IRCampaignData.Builder campaignInfo = new IRCampaignData.Builder();
campaignInfo.setCampaignID(campaignID);
campaignInfo.setTemplateID(0);
campaignInfo.build();
InviteReferralsApi.getInstance(activityContext).getSharingDetails(new InviteReferralsSharingInterface() {
@Override
public void getShareData(JSONObject sharingDetails) {
//do your task here
}
}, userInfo, campaignInfo);
val userData = IRUserData.Builder();
userData.setUserName(name);
userData.setUserEmail(email);
userData.setUserMobile(mobile);
userData.setCustomValueOne(null);
userData.setCustomValueTwo(null);
userData.build();
val campaignInfo = IRCampaignData.Builder();
campaignInfo.setCampaignID(campaignID);
campaignInfo.setTemplateID(0);
campaignInfo.build();
InviteReferralsApi.getInstance(activityContext).getSharingDetails(object : InviteReferralsSharingInterface {
override fun getShareData(p0: JSONObject?) {
//do your task here
}
}, userData, campaignInfo)
NOTE
If you don't pass user data then this callback will provide you with only the campaign-related data. However, if you pass user data then it will provide all data i.e. campaign data along with the user data.
Response with User Data
{
"Authentication":"success",
"CustomerName":"Jack90",
"Email":"[email protected]",
"referral_code":"JACK",
"referral_link":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972",
"unique_code":"",
"stats":{
"totalInvites":"8",
"totalClicks":"234",
"totalConverts":"0"
},
"campaignID":200,
"popup_shareText":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972 Refer and win #Referral #Contests #inviteReferrals",
"shareSubject":"Explore Tagnpin Referral Contest",
"inviteMail":"Hi, Your friend Jack90, has invited you to try Tagnpin through his referral link.\nAccept the invitation by clicking the link mentioned below.\nhttps:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972",
"twitter_share_text":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972 Refer and win #Referral #Contests #inviteReferrals",
"email_shareText":"Hi, Your friend Jack90, has invited you to try Tagnpin through his referral link.\nAccept the invitation by clicking the link mentioned below.\nhttps:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972",
"whatsapp_shareText":"Refer and win #Referral #Contests #inviteReferrals https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972",
"sms_shareText":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972 Refer and win #Referral #Contests #inviteReferrals",
"twitter_shareText":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972 Refer and win #Referral #Contests #inviteReferrals",
"linkedin_shareText":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972 Refer and win #Referral #Contests #inviteReferrals",
"pinterest_shareText":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972 Refer and win #Referral #Contests #inviteReferrals",
"fb_shareText":{
"fbLink":"https:\/\/tagnpinyA.talentfizz.com\/c\/i\/200\/972 Refer and win #Referral #Contests #inviteReferrals",
"fbImage":"https:\/\/s3.ap-south-1.amazonaws.com\/stage-tagnpin\/images\/fb_49_200.jpg"
},
"loginScreenData":"<link rel=\"stylesheet\" href=\"https:\/\/maxcdn.bootstrapcdn.com\/bootstrap\/3.3.5\/css\/bootstrap.min.css\"><style>body{background: transparent;}<\/style><div class=\"text-center\">\n <h3><br \/>Heading<\/h3>\n <p class=\"lead\">\n The description of your referral campaign \n <br>\n comes here\n <\/p>\n<\/div>",
"shareScreenData":"<link rel=\"stylesheet\" href=\"https:\/\/maxcdn.bootstrapcdn.com\/bootstrap\/3.3.5\/css\/bootstrap.min.css\"><style>body{background: transparent;}<\/style><div class=\"text-center\">\n<h3><br \/>Heading<\/h3>\n<p class=\"lead\">The description of your referral campaign <br \/>comes here<br \/><span style=\"color: #ba372a;\"><strong>I am from Campaign : 200<\/strong><\/span><\/p>\n<\/div>",
"howItWorks":"<div><img src=\"https:\/\/as2.ftcdn.net\/v2\/jpg\/01\/87\/31\/25\/1000_F_187312561_kMY7AYVLSwCz6Jua7KusMMPQtb4TayFA.jpg\" width=\"500\" height=\"500\" alt=\"\" style=\"display: block; margin-left: auto; margin-right: auto;\" \/><\/div>",
"tnc":"This is Terms and Conditions section."
}
Response without User Data
{
"Authentication":"success",
"campaignID":200,
"popup_shareText":"{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"shareSubject":"Explore Tagnpin Referral Contest",
"inviteMail":"Hi, Your friend {customer name}, has invited you to try Tagnpin through his referral link.\nAccept the invitation by clicking the link mentioned below.\n{referral link}",
"twitter_share_text":"{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"email_shareText":"Hi, Your friend {customer name}, has invited you to try Tagnpin through his referral link.\nAccept the invitation by clicking the link mentioned below.\n{referral link}",
"whatsapp_shareText":"Refer and win #Referral #Contests #inviteReferrals ",
"sms_shareText":"{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"twitter_shareText":"{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"linkedin_shareText":"{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"pinterest_shareText":"{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"fb_shareText":{
"fbLink":"{referral link} Refer and win #Referral #Contests #inviteReferrals ",
"fbImage":"https:\/\/s3.ap-south-1.amazonaws.com\/stage-tagnpin\/images\/fb_49_200.jpg"
},
"loginScreenData":"<link rel=\"stylesheet\" href=\"https:\/\/maxcdn.bootstrapcdn.com\/bootstrap\/3.3.5\/css\/bootstrap.min.css\"><style>body{background: transparent;}<\/style><div class=\"text-center\">\n <h3><br \/>Heading<\/h3>\n <p class=\"lead\">\n The description of your referral campaign \n <br>\n comes here\n <\/p>\n<\/div>",
"shareScreenData":"<link rel=\"stylesheet\" href=\"https:\/\/maxcdn.bootstrapcdn.com\/bootstrap\/3.3.5\/css\/bootstrap.min.css\"><style>body{background: transparent;}<\/style><div class=\"text-center\">\n<h3><br \/>Heading<\/h3>\n<p class=\"lead\">The description of your referral campaign <br \/>comes here<br \/><span style=\"color: #ba372a;\"><strong>I am from Campaign : 200<\/strong><\/span><\/p>\n<\/div>",
"howItWorks":"<div><img src=\"https:\/\/as2.ftcdn.net\/v2\/jpg\/01\/87\/31\/25\/1000_F_187312561_kMY7AYVLSwCz6Jua7KusMMPQtb4TayFA.jpg\" width=\"500\" height=\"500\" alt=\"\" style=\"display: block; margin-left: auto; margin-right: auto;\" \/><\/div>",
"tnc":"This is Terms and Conditions section."
}
Track Invites Manually
Implement this function if you have created your own sharing screen and you want to collect stats in case a customer clicks on any sharing icon.
InviteReferralsApi.getInstance(activityContext).trackInvite(sourceName, campaignID);
InviteReferralsApi.getInstance(activityContext).trackInvite(sourceName, campaignID)
- ‘sourceName’ parameter will be as per the app name.
- ‘campaignID’ parameter will be the ID of the campaign against which you want to increase invites.
Below are the details: -
App Name | Source Name |
---|---|
Gmail | |
Messenger | messenger or fb message |
facebook or fbshare | |
tweet or twitter | |
Linked in | |
SMS | sms |
Telegram | telegram |
Referral Link | referral_link_invites_app |
Updated over 1 year ago