Native SDK
Integration for Native SDK
- Demo App with SDK can be downloaded from here
Follow the steps below:
1) Add the following repository to your project-level build.gradle
file:
build.gradle
file:repositories {
mavenCentral()
google()
}
- If you have upgraded your app to AndroidX, then add the following dependency to your app’s
build.gradle
file:
dependencies {
implementation 'com.android.installreferrer:installreferrer:2.1'
implementation 'com.invitereferrals.invitereferrals:invitereferrals:v1.0.12'
}
OR
- If your app is not upgraded to AndroidX for now, then add the following dependency to your app’s
build.gradle
file:
dependencies {
implementation 'com.android.installreferrer:installreferrer:2.1'
implementation 'com.invitereferrals.invitereferrals:invitereferrals:v28-4.3.8'
}
2) Configure AndroidManifest.xml
file of your app
AndroidManifest.xml
file of your app<manifest>
.......
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/>
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<application>
........
<meta-data android:name="invitereferrals_bid" android:value="29XX" />
<meta-data android:name="invitereferrals_bid_e" android:value="DD85448BF2678A482D5XXXX8425AAC6F"/>
</application>
</manifest
Important:
In above example Dummy Brand ID and Encryption keys shown. Kindly login your IR_account to see your credentials.
- If you want to use contact_sync feature, then add the following permissions into your apps'
AndroidManifest.xml
.
<manifest>
<uses-permission android:name="android.permission.READ_CONTACTS" />
<uses-permission android:name="android.permission.WRITE_CONTACTS" />
</manifest>
3) SDK Initialization
- If you don’t have your own application class, then in the
manifest.xml
file register InviteReferrals' Application class:
<application android:name="com.invitereferrals.invitereferrals.InviteReferralsApplication">
........
........
</application>
OR
- If you have your own application class then inside your Application class'
onCreate()
method, insert the below code:
@Override
public void onCreate() {
super.onCreate();
.....
InviteReferralsApplication.register(this);
.....
}
4) Pass User Details for Auto-Login (Single Sign In)
InviteReferralsApi.getInstance(getActivity()).userDetails((String)name, (String)email, (String)mobile, campaignID(int), (String)subscriptionID, (String)customValues);
For Example,
InviteReferralsApi.getInstance(getActivity()).userDetails('Tom', '[email protected]', '9999999999', 0, null, null);
To get UserDetails method Response
as callback
, use the below code:
InviteReferralsApi.getInstance(getActivity()).userDetailListener(new UserDetailsCallback() {
@Override
public void userDetails(JSONObject ApiResponse) {
Log.e("Users","Response = " + ApiResponse);
}
});
5) Add Referral Button
InviteReferralsApi.getInstance(getActivity()).userDetails((String)name, (String)email, (String)mobile, campaignID(int), (String)subscriptionID, (String)customValues);
- Add the following line in your custom button click. Replace
CampaignID
with the id of the referral campaign.
Info:
Default campaignID will be applied if no campaignID specified.
InviteReferralsApi.getInstance(getActivity()).inline_btn((int)CampaignID);
For Example,
//For specified campaign
InviteReferralsApi.getInstance(getActivity()).inline_btn(1765);
//For Default Campaign you can pass 0 as CampaignID:
InviteReferralsApi.getInstance(getActivity()).inline_btn(0);
6) Show Refer a friend Popup
Just add the following line in the activity in which you want to show the referral program popup.
InviteReferralsApi.getInstance(getActivity()).invite((String)CUSTOM_RULE);
For Example,
InviteReferralsApi.getInstance(getActivity()).invite("home");
Info:
You may then set rules in the InviteReferrals Campaign Settings to show campaign popup after specific time delay or after specific number of App launches on this view.
7) Track Install / Register / Sale Events
This is how you call tracking()
method to track:
Install Event
InviteReferralsApi.getInstance(getActivity()).tracking("install", null, 0, null, null);
Register Event
InviteReferralsApi.getInstance(getActivity()).tracking("register", (String)emailID, (int)purchaseValue, (String)referCode, (String)unique_code);
Sale Event
InviteReferralsApi.getInstance(getActivity()).tracking("sale", (String)orderID, (int)purchaseValue, (String)referCode, (String)uniqueCode);
- To get
Tracking method Response
ascallback
, use the below code:
InviteReferralsApi.getInstance(getActivity()).ir_TrackingCallbackListener(new IRTrackingCallback() {
@Override
public void ir_trackingCallbackForEventName(JSONObject tracking_response) {
Log.e("Tracking","Response = " + tracking_response);
}
});
8) Show Welcome Message
Show welcome message to the customer, if he comes through the referral of his friend.
InviteReferralsApi.getInstance(getActivity()).showWelcomeMessage();
FAQs:
Q1. How to set Background for InviteReferrals Screens?
Ans. You can also set background of your choice for Invitereferrals screens by passing the below KEY in your app's strings.xml file:
<string name= "ir_screenBackgroundImg">#FFFFFF</string>
- Various Values can be passed here for setting background.
a) Drawable Image Name. [ Note:- Image should be available in your app's drawable folder]
<string name= "ir_screenBackgroundImg">green_wallpaper</string>
b) Image URL. [ Note:- File extension should be '.jpg' or '.jpeg' or '.png']
<string name= "ir_screenBackgroundImg">https://lh3.googleusercontent.com/-MMnX4mZQ6o0/XmoCW5D51JI/AAAAAAAACjc/VaO9kIshx5UOFVyKlrbRpGNomI1Hj8xngCK8BGAsYHg/s512/2020-03-12.jpg </string>
c) RGBA Color Format.
<string name= "ir_screenBackgroundImg">rgba(250,56,78,1)</string> //RED Color
d) HEX Color Code.
<string name= "ir_screenBackgroundImg">#FF6699</string> //PINK Color
Info:
If no VALUE is passed, then it will automatically use Default Color ie., WHITE Color.
Q2. How to set custom fonts ?
Ans. You can use Custom fonts for InviteReferrals Screens texts to match with your App’s text fonts.
To do this, add fonts.ttf
files inside _app__→src→main→_asset→ fonts folder to your Android project. And also add this file name in the KEY in your app's strings.xml
file.
For example:- "DroidSans-Bold.ttf".
<string name= "ir_fontStyle">fonts/DroidSans-Bold.ttf</string>
Q3. How can I change sharing button icons like facebook, whatsapp in Sharing Screen of Invitereferrals ?
Ans. You can change icons of sharing options visible on sharing screen by putting your icon images into your apps' drawable
folder with the following names for the respective apps.
App Name | Drawable Icon Name |
---|---|
ir_whatsapp | |
Gmail | ir_gmail |
Messenger | ir_messenger |
ir_facebook | |
ir_twitter | |
ir_linked_in | |
SMS | ir_sms |
Google + | ir_google_plus |
ir_pinterest | |
Telegram | ir_telegram |
More option (right side icon in referral link box) | more_icon |
Q4. How can I perform a custom action on Done/Close Button Click of InviteReferrals Screens ?
Ans. For adding custom action on Done/Close Button click, add below listener:
InviteReferralsApi.getInstance(getActivity()).closeButtonListener(new ir_CloseButtonCallbackInterface() {
@Override
public void HandleDoneButtonAction {
// Do Your stuff here …………..
}
});
Q5. How to translate InviteReferrals Screen into localize language as per default language of Android device?
Ans. For Localize language such as Vietnamese follow the following steps:
a) Select your Project Module on the left of Android Panel. Select Module Name and choose Values _directory under the _Resource subsection.
b) Right-click on Values directory and add a new values resource file. Then you will see a screen like this:
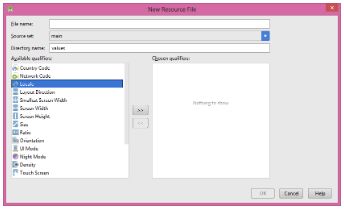
c) Enter file name as strings.xml
.
Warning:
strings.xml
is the default filename android uses for localized text. Resist the urge to name the file something else, otherwise you will have to type the name of your .xml file every time you reference a localized string.
d) Select Locale option under the Available qualifiers tag. Then click on the button with double-right angular bracket symbol.
e) Then select the required language from the available languages under _Language _tag. And finally click Ok button.
f) Select strings.xml
(English) and replace its contents with the following:
<string name="app_name">invitereferrals</string>
<string name="more_options">More options to share</string>
<string name="stats">My Referral Statistics</string>
<string name="tnc">Terms And Conditions</string>
<string name="how_it_works">How It Works</string>
<string name="ir_login_userNameHint">Enter Name</string>
<string name="ir_login_userEmailHint">Enter Email Address</string>
<string name="ir_login_userMobileHint">%d Digit Mobile Number</string>
<string name="ir_login_buttonText">Register</string>
<string name="loading_message">Please wait. Page is loading...</string>
<string name="valid_email">Enter Valid Email ID</string>
<string name="Invalid_mobie">Enter Mobile Number</string>
<string name="err_msg">Something went wrong</string>
<string name="ir_actionbarLoginScreenTitle">Login Screen</string>
<string name="ir_actionbarShareScreenTitle">Sharing Screen</string>
<string name="ir_actionbarBackground">#a25016</string>
<string name="ir_actionbarTitleColor">rgba(165,188,210,1)</string>
<string name="ir_actionbarButtonTitle">close</string>
<string name="ir_btnTintColor">#56C13E</string>
g) To change the text for Vietnamese, select strings.xml
(Vietnamese) and replace its contents with the following:
<string name="app_name">invitereferrals</string>
<string name="more_options">Nhiều tùy chọn để chia sẻ</string>
<string name="stats">Thống kê giới thiệu của tôi</string>
<string name="tnc">Các điều khoản và điều kiện</string>
<string name="how_it_works">Làm thế nào nó hoạt động</string>
<string name="ir_login_userNameHint">Tên</string>
<string name="ir_login_userEmailHint">E-mail</string>
<string name="ir_login_userMobileHint">Số di động Số %d</string>
<string name="ir_login_buttonText">Ghi danh</string>
<string name="valid_email">Nhập ID Email hợp lệ</string>
<string name="err_msg">Đã xảy ra lỗi</string>
<string name="loading_message">Xin chờ. Đang tải trang...</string>
<string name="ir_actionbarLogScreenTitle">Màn hình đăng nhập</string>
<string name="ir_actionbarShareScreenTitle">Màn hình chia sẻ</string>
<string name="ir_actionbarBackground">#a25016</string>
<string name="ir_actionbarTitleColor">rgba(165,188,210,1)</string>
<string name="ir_actionbarButtonTitle">gần</string>
<string name="ir_btnTintColor">#56C13E</string>
Updated about 3 years ago